实体类:
public class Student
{
public int Id { get; set; }
public string Name { get; set; }
public string[] MultipleCareers { get; set; }
public Location Location { get; set; }
}
public class Location
{
public int Row { get; set; }
public int Col { get; set; }
}
Program.cs代码:
class Program
{
private static void Main(string[] args)
{
object student = new Student
{
Id = 1,
Name = "zhang san",
MultipleCareers =new string[]{"教师","程序员","作家","画家"},
Location = new Location
{
Row = 10,
Col = 20
}
};
VisitProperties<Student>(student);
}
private static void VisitProperties<T>(object obj)
{
Type type = obj.GetType();
ParameterExpression paraExpression = Expression.Parameter(typeof (T), "object");
foreach (PropertyInfo prop in type.GetProperties())
{
Type propType = prop.PropertyType;
if (propType.IsPrimitive || propType == typeof (String))
{
VisitProperty<T>(obj, prop, paraExpression, paraExpression);
}
else
{
Console.WriteLine("not primitive property: " + prop.Name);
Type otherType = prop.PropertyType;
MemberExpression memberExpression = Expression.Property(paraExpression, prop);
foreach (PropertyInfo otherProp in otherType.GetProperties())
{
VisitProperty<T>(obj, otherProp, memberExpression, paraExpression);
}
}
Console.WriteLine("--------------------------------");
}
}
private static void VisitProperty<T>(Object obj, PropertyInfo prop, Expression instanceExpression, ParameterExpression parameterExpression)
{
Console.WriteLine("property name: " + prop.Name);
MemberExpression memExpression = Expression.Property(instanceExpression, prop);
Expression objectExpression = Expression.Convert(memExpression, typeof (object));
Expression<Func<T, object>> lambdaExpression = Expression.Lambda<Func<T, object>>(objectExpression,
parameterExpression);
Console.WriteLine("expression tree: " + lambdaExpression);
Func<T, object> func = lambdaExpression.Compile();
Console.WriteLine("value: " + func((T) obj));
}
}
运行结果如图:
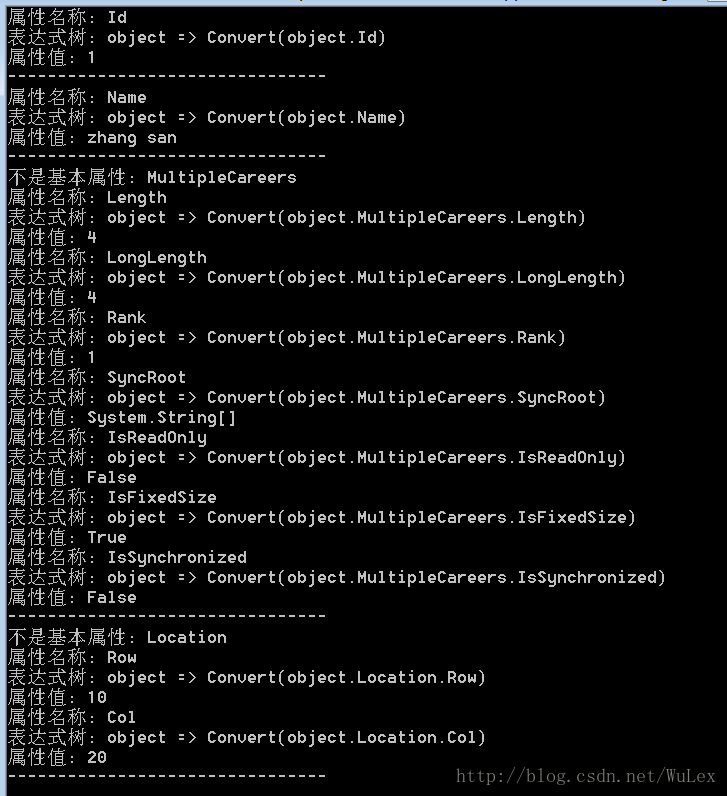