带来混沌的是无知而不是知识
FileInfo.cs实体类
public class FileInfo
{
public string Name { get; set; }
public int ServerID { get; set; }
}
ServerInfo.cs实体类
public class ServerInfo
{
public int ServerID { get; set; }
public string ServerIP { get; set; }
public bool Status { get; set; }
}
DFSEntities.cs数据库上下文
public class DFSEntities:DbContext
{
protected override void OnModelCreating(DbModelBuilder modelBuilder)
{
modelBuilder.Entity<FileInfo>().ToTable("FileInfo")
.HasKey(m => m.Name);
modelBuilder.Entity<ServerInfo>().ToTable("ServerInfo")
.HasKey(m => m.ServerID);
base.OnModelCreating(modelBuilder);
}
public virtual DbSet<FileInfo> FileInfo { get; set; }
public virtual DbSet<ServerInfo> ServerInfo { get; set; }
}
Index.aspx内容
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<meta http-equiv="Content-Type" content="text/html; charset=utf-8"/>
<title></title>
</head>
<body>
<form id="form1" runat="server" method="post" action="FileUpload.ashx" enctype="multipart/form-data">
<div>
<asp:FileUpload ID="FileUpload1" runat="server" />
<input type="submit" value="Submit" />
</div>
</form>
</body>
</html>
FileUpload.ashx代码
public class FileUpload : IHttpHandler
{
public void ProcessRequest(HttpContext context)
{
context.Response.ContentType = "text/plain";
var file = context.Request.Files["FileUpload1"];
if(file != null)
{
using(DFSEntities dfs = new DFSEntities())
{
var serverList = dfs.ServerInfo.Where(s => s.Status).ToList();
int n = serverList.Count;
int r = new Random().Next();
int i = r % n;
ServerInfo server = serverList[i];
string address = string.Format("http://{0}/SaveFile.ashx",server.ServerIP);
HttpClient client = new HttpClient();
var content = new MultipartFormDataContent();
string fileName = Guid.NewGuid() + Path.GetExtension(file.FileName);
content.Add(new StreamContent(file.InputStream), "file", fileName);
content.Add(new StringContent(server.ServerID.ToString()), "serverID");
var hrm = client.PostAsync(address, content).Result;
context.Response.Write(hrm.Content.ReadAsStringAsync().Result);
return;
}
}
context.Response.Write("{status:0,notice:\"未找到key=file的文件。\"}");
}
public bool IsReusable
{
get { return false; }
}
}
SaveFile.ashx代码:
public class SaveFile : IHttpHandler
{
static readonly string ServerIP = ConfigurationManager.AppSettings["ServerIP"];
public void ProcessRequest(HttpContext context)
{
context.Response.ContentType = "text/plain";
var file = context.Request.Files["file"];
string sid = context.Request.Form["serverID"];
int serverID = 0;
if(int.TryParse(sid, out serverID) && file != null)
{
DateTime now = DateTime.Now;
string dir =String.Format("/Files/{0}/{1}/{2}/{3}/",now.Year,now.Month,now.Day,now.Hour);
string absPath = context.Server.MapPath(dir);
if(!Directory.Exists(absPath))
Directory.CreateDirectory(absPath);
string guid = Guid.NewGuid().ToString();
string name = guid + Path.GetExtension(file.FileName);
string fullPath = String.Format("{0}\\{1}",absPath,name);
string returnUrl = ServerIP + dir + name;
file.SaveAs(fullPath);
using(DFSEntities dfs = new DFSEntities())
{
dfs.FileInfo.Add(new EntityFramework.FileInfo
{
Name = returnUrl,
ServerID = serverID
});
if(dfs.SaveChanges() > 0)
{
context.Response.Write(String.Format("{{status:1,notice:\"上传成功\",data:\"{0}\"}}", returnUrl));
return;
}
}
context.Response.Write("{status:0,notice:\"上传失败\"}");
return;
}
context.Response.Write("{status:0,notice:\"上传失败\"}");
}
public bool IsReusable
{
get { return false; }
}
}
sql脚本:
SET ANSI_NULLS ON
GO
SET QUOTED_IDENTIFIER ON
GO
SET ANSI_PADDING ON
GO
CREATE TABLE [dbo].[ServerInfo](
[ServerID] [int] IDENTITY(1,1) NOT NULL,
[ServerIP] [varchar](50) NOT NULL,
[Status] [bit] NOT NULL,
CONSTRAINT [PK_ServerInfo] PRIMARY KEY CLUSTERED
(
[ServerID] ASC
)WITH (PAD_INDEX = OFF, STATISTICS_NORECOMPUTE = OFF, IGNORE_DUP_KEY = OFF, ALLOW_ROW_LOCKS = ON, ALLOW_PAGE_LOCKS = ON) ON [PRIMARY]
) ON [PRIMARY]
GO
SET ANSI_PADDING OFF
GO
SET IDENTITY_INSERT [dbo].[ServerInfo] ON
INSERT [dbo].[ServerInfo] ([ServerID], [ServerIP], [Status]) VALUES (1, N'192.168.191.1', 0)
INSERT [dbo].[ServerInfo] ([ServerID], [ServerIP], [Status]) VALUES (2, N'localhost:55424', 1)
SET IDENTITY_INSERT [dbo].[ServerInfo] OFF
USE [master]
GO
ALTER DATABASE [SAMPLEDB] SET READ_WRITE
GO
运行结果如图:

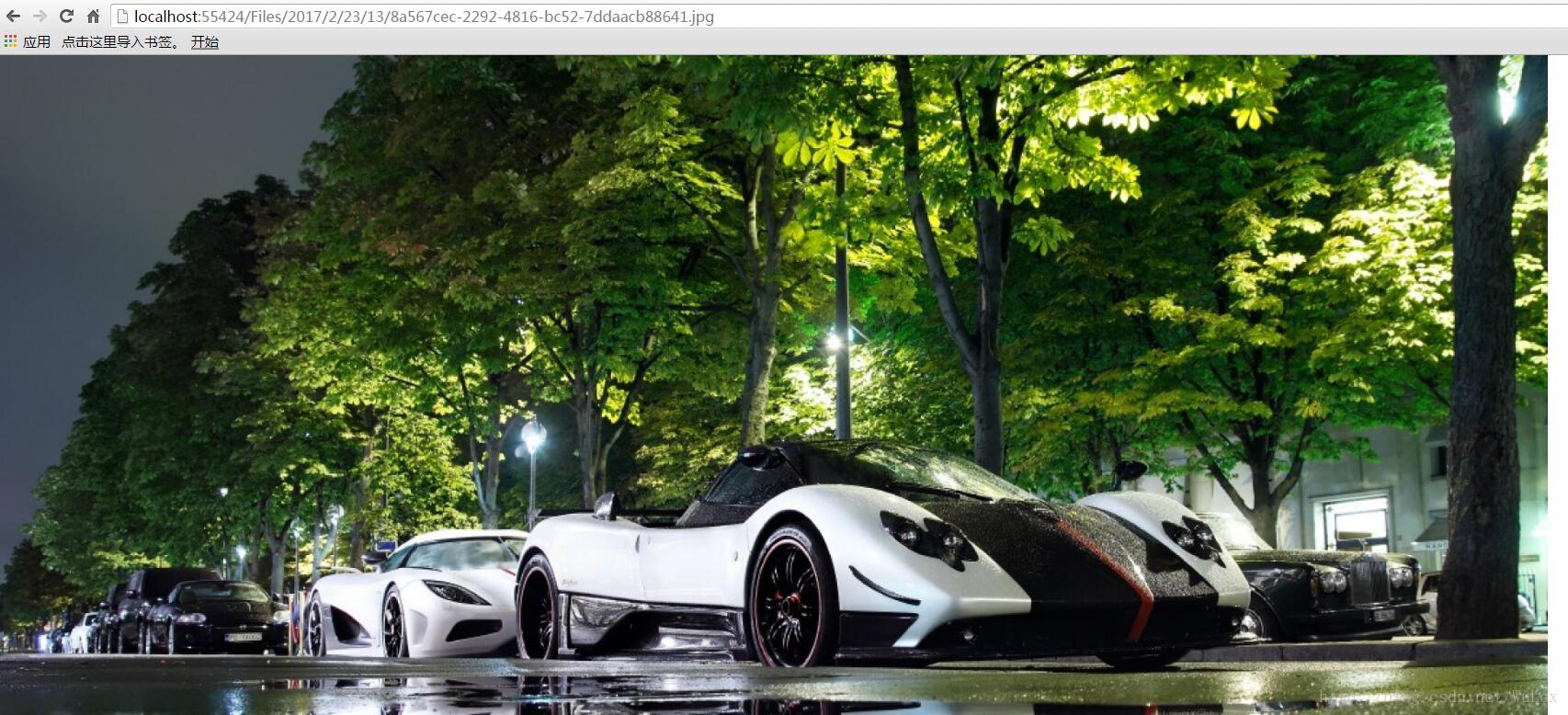